Android Studio 12일차
2021. 11. 16. 17:10ㆍAndroid Development
가수 그룹 관리 DB만들기
더보기
DB browser 다운로드.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="4dp"
android:orientation="horizontal">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="이름" />
<EditText
android:id="@+id/etName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:ems="10"
android:inputType="textPersonName" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="4dp"
android:orientation="horizontal">
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="인원" />
<EditText
android:id="@+id/etNumber"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:ems="10"
android:inputType="number" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/btInit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:layout_weight="1"
android:text="초기화" />
<Button
android:id="@+id/btInsert"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:layout_weight="1"
android:text="입력" />
<Button
android:id="@+id/btSelect"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:layout_weight="1"
android:text="조회" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<EditText
android:id="@+id/etNameResult"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="1"
android:lines="10"/>
<EditText
android:id="@+id/etNumberResult"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:background="@color/teal_200"
android:layout_weight="1"
android:lines="10" />
</LinearLayout>
</LinearLayout>
레이아웃
package com.example.test27;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.database.Cursor;
import android.database.DatabaseErrorHandler;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
myDBHelper myHelper;
EditText edtName, edtNumber, edtNameResult, edtNumberResult;
Button btnInit, btnInsert, btnSelect;
SQLiteDatabase sqlDB;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
setTitle("가수 그룹 관리 DB");
edtName = findViewById(R.id.etName);
edtNumber = findViewById(R.id.etNumber);
edtNameResult = findViewById(R.id.etNameResult);
edtNumberResult = findViewById(R.id.etNumberResult);
btnInit = findViewById(R.id.btInit);
btnInsert = findViewById(R.id.btInsert);
btnSelect = findViewById(R.id.btSelect);
myHelper = new myDBHelper(this);
btnInit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
sqlDB = myHelper.getWritableDatabase();
myHelper.onUpgrade(sqlDB, 1, 2);
sqlDB.close();
}
});
btnInsert.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
sqlDB = myHelper.getWritableDatabase();
sqlDB.execSQL("INSERT INTO groupTBL VALUES('" + edtName.getText().toString() + "'," + edtNumber.getText().toString() + ")");
sqlDB.close();
Toast.makeText(getApplicationContext(), "입력됨", Toast.LENGTH_SHORT).show();
}
});
btnSelect.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
sqlDB = myHelper.getReadableDatabase();
Cursor cursor;
cursor = sqlDB.rawQuery("SELECT * FROM groupTBL;", null);
String strNames = "그룹이름" + "\r\n" + "------" + "\r\n";
String strNumbers = "인원" + "\r\n" + "------" + "\r\n";
while (cursor.moveToNext()) {
strNames += cursor.getString(0) + "\r\n";
strNumbers += cursor.getString(1) + "\r\n";
}
edtNameResult.setText(strNames);
edtNumberResult.setText(strNumbers);
cursor.close();
sqlDB.close();
}
});
}
public class myDBHelper extends SQLiteOpenHelper {
public myDBHelper(@Nullable Context context) {
super(context, "groupDB", null, 1);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL("CREATE TABLE groupTBL ( gName CHAR(20) PRIMARY KEY" + ", gNumber INTEGER);");
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS groupTBL");
onCreate(db);
}
}
}
MainActivity
갤러리 영화 포스터 만들기.
더보기
package com.example.test27;
import androidx.activity.result.ActivityResult;
import androidx.activity.result.ActivityResultCallback;
import androidx.activity.result.ActivityResultLauncher;
import androidx.activity.result.contract.ActivityResultContracts;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AlertDialog;
import androidx.appcompat.app.AppCompatActivity;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.BlurMaskFilter;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.EmbossMaskFilter;
import android.graphics.Paint;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.MotionEvent;
import android.view.View;
import android.view.ViewGroup;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.BaseAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Gallery;
import android.widget.GridView;
import android.widget.ImageButton;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.ListView;
import android.widget.Toast;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
setTitle("갤러리 영화 포스터");
Gallery gallery = findViewById(R.id.gallery1);
// 동작이 안 되는 것은 아니지만, 앞으로 사용을 비추함.
MyGalleryAdapter galAdapter = new MyGalleryAdapter(this);
gallery.setAdapter(galAdapter);
}
public class MyGalleryAdapter extends BaseAdapter {
// 멤버 변수
Context context;
// 이미지 아이디
Integer[] posterID = {R.drawable.mov11, R.drawable.mov12,
R.drawable.mov13, R.drawable.mov14, R.drawable.mov15,
R.drawable.mov16, R.drawable.mov17, R.drawable.mov18,
R.drawable.mov19, R.drawable.mov20};
// 생성자
public MyGalleryAdapter(Context c) {
context = c;
}
@Override
public int getCount() {
return posterID.length;
}
@Override
public Object getItem(int position) {
return null;
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View convertView, ViewGroup
parent) {
ImageView imageview = new ImageView(context);
imageview.setLayoutParams(new Gallery.LayoutParams(200, 300));
imageview.setScaleType(ImageView.ScaleType.FIT_CENTER);
imageview.setPadding(5, 5, 5, 5);
imageview.setImageResource(posterID[position]);
imageview.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
ImageView ivPoster = findViewById(R.id.ivPoster);
ivPoster.setScaleType(ImageView.ScaleType.FIT_CENTER);
ivPoster.setImageResource(posterID[position]);
}
});
return imageview;
}
}
}
미디어 플레이어 예시
더보기
package com.example.test27;
import android.media.MediaPlayer;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ProgressBar;
import android.widget.SeekBar;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ProgressBar pb1;
Button btnInc, btnDec;
pb1 = findViewById(R.id.progressBar);
btnInc = findViewById(R.id.btInc);
btnDec = findViewById(R.id.btDec);
TextView tvSeek = findViewById(R.id.tvSeek);
SeekBar seekbar1 = findViewById(R.id.seekBar);
btnInc.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
pb1.incrementProgressBy(10);
}
});
btnDec.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
pb1.incrementProgressBy(-10);
}
});
seekbar1.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) {
//시크바가 변화 한다면
tvSeek.setText("진행률 : " + progress + " %");
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
//시크바를 간주 점프 시작.
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
//시크바 간주 점프 동작 마침.
}
});
}
}
시크바 사용 예시
더보기
import android.os.Bundle;
import android.os.SystemClock;
import android.view.View;
import android.widget.Button;
import android.widget.ProgressBar;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ProgressBar pb1 = findViewById(R.id.seekBar2);
ProgressBar pb2 = findViewById(R.id.seekBar3);
Button bt= findViewById(R.id.button6);
bt.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
for (int i = 0; i < 100; i++) {
pb1.setProgress(pb1.getProgress() + 2);
pb2.setProgress(pb2.getProgress() + 1);
SystemClock.sleep(100);
}
}
});
}
}
스레드를 이용한 프로그레스바 사용 예시
더보기
import android.os.Bundle;
import android.os.SystemClock;
import android.view.View;
import android.widget.Button;
import android.widget.ProgressBar;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button bt = findViewById(R.id.bt);
ProgressBar pb1,pb2;
pb1 = findViewById(R.id.pb1);
pb2 = findViewById(R.id.pb2);
TextView tv1 = findViewById(R.id.tv1);
TextView tv2 =findViewById(R.id.tv2);
bt.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
new Thread(){
@Override
public void run() {
// super.run();
for (int i = pb1.getProgress(); i < 100; i = i+2){
runOnUiThread(new Runnable() {
@Override
public void run() {
pb1.setProgress(pb1.getProgress() + 2);
tv1.setText("1번 진행률" + pb1.getProgress() + "%");
}
});
SystemClock.sleep(100);
}
}
}.start();
new Thread(){
// @Override
public void run() {
// super.run();
for (int i = pb2.getProgress(); i < 100; i = i++){
runOnUiThread(new Runnable() {
@Override
public void run() {
pb2.setProgress(pb2.getProgress() + 1);
tv2.setText("2번 진행률" + pb2.getProgress() + "%");
}
});
SystemClock.sleep(100);
}
}
}.start();
}
});
}
}
안드로이드 스튜디오 에서 구글 지도 이용해보기
더보기
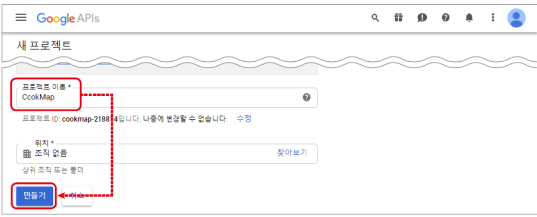


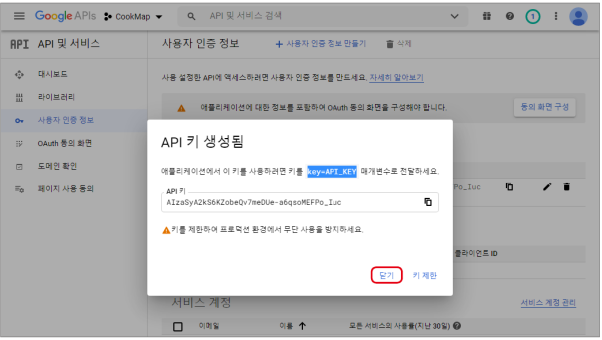

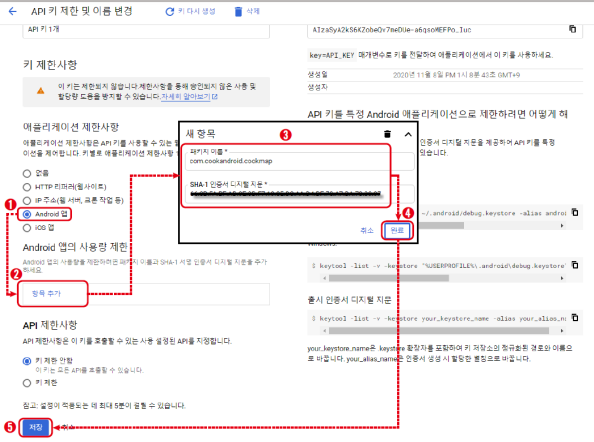
// 아래 디지털 지문 생성은 파워셀은 안되고 명령 프롬프트에서 처리 바랍니다.
keytool -list -v -alias androiddebugkey -keystore %USERPROFILE%\.android\debug.keystore
비번 : android
https://console.cloud.google.com/apis/library/maps-android-backend.googleapis.com
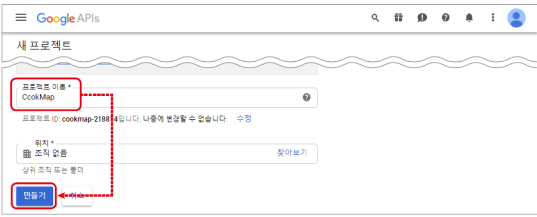


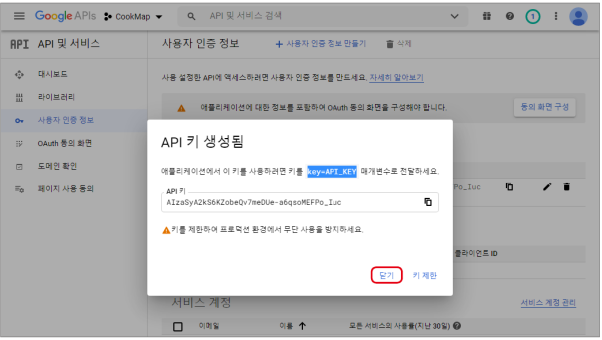

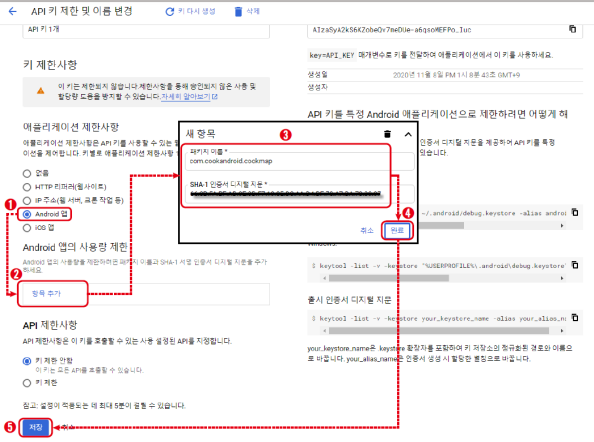
android studio 내의 sdk manager 에서 google play service 설치
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.test27">
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="com.google.android.providers.gsf.permission.READ_GSERVICES" />
<meta-data
android:name="com.google.android.gms.version"
android:value="@integer/google_play_services_version" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.Test27">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<meta-data
android:name="com.google.android.maps.v2.API_KEY"
android:value="받은 API값 입력" />
<uses-library
android:name="org.apache.http.legacy"
android:required="false" />
</application>
</manifest>
'Android Development' 카테고리의 다른 글
Android semiProject (0) | 2021.11.24 |
---|---|
Android Studio 13일차 (0) | 2021.11.17 |
Android Studio 11일차 (0) | 2021.11.15 |
Android Studio 10일차 (0) | 2021.11.12 |
Android Studio 9일차 (0) | 2021.11.11 |